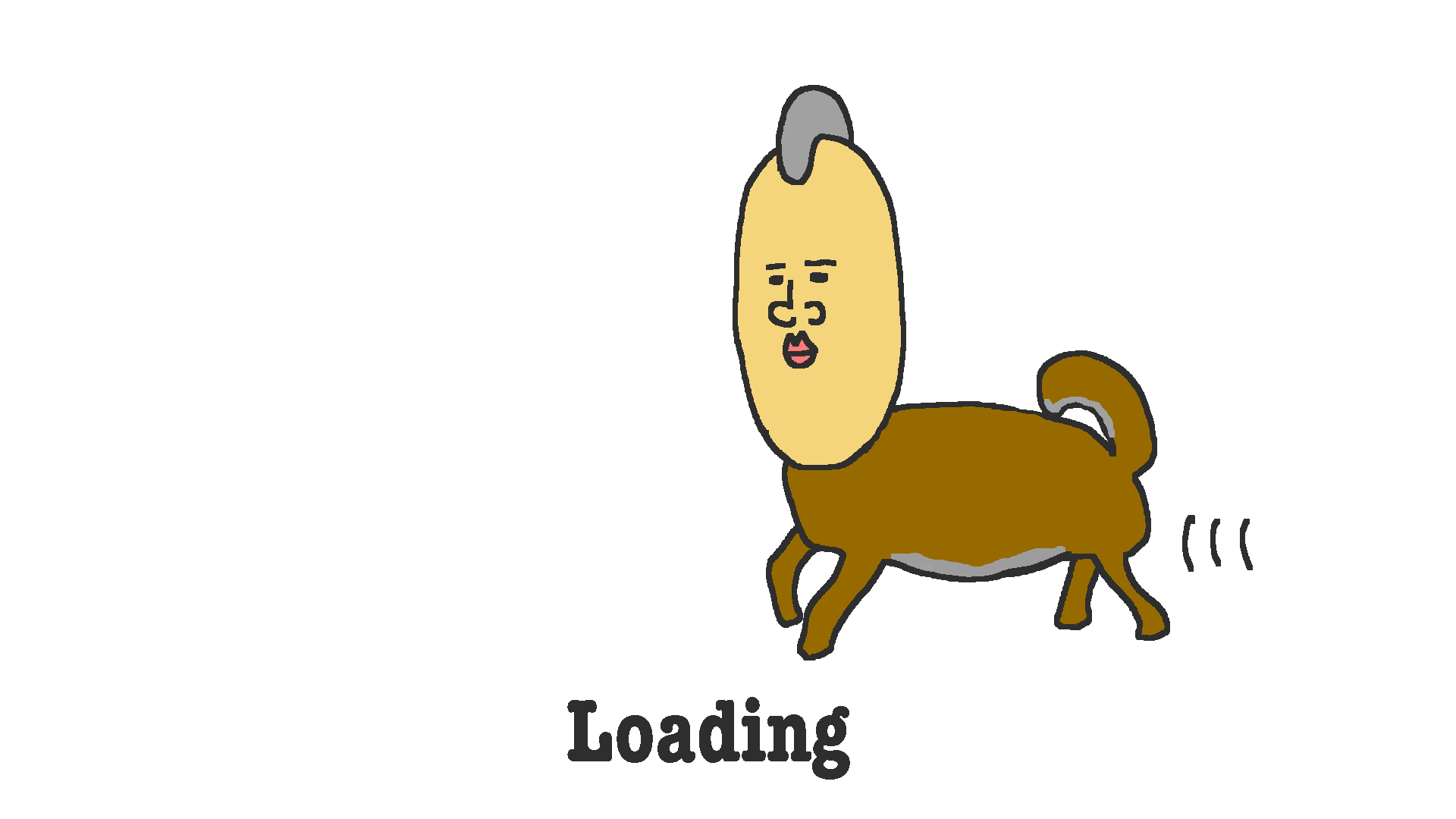
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
$('#get_template').on('click', function (e) {
e.preventDefault();
$.ajax({
url: '/table',
type: 'GET',
dataType: 'json',
data: {
count: $('input[name="count"]').val()
},
success: function (data) {
// 👇 jqueryでtableを作成して描画する処理
let table = $('<table>', {});
let thead = $('<thead>', {});
let thead_tr = $('<tr>', {});
let th1 = $('<th>', {
text: 'Id'
});
thead_tr.append(th1);
let th2 = $('<th>', {
text: 'Name'
});
thead_tr.append(th2);
thead.append(thead_tr);
table.append(thead);
let tbody = $('<tbody>', {});
Object.keys(data.users).forEach(function (key) {
let tbody_tr = $('<tr>', {});
let td1 = $('<td>', {
text: data.users[key].id
});
tbody_tr.append(td1);
let td2 = $('<td>', {
text: data.users[key].name
});
tbody_tr.append(td2);
tbody.append(tbody_tr);
});
table.append(tbody);
$('#template_area').html(table);
},
error: function (error) {
console.log(error);
}
});
});
📁 src/app/Http/Controllers/WelcomeController.php
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class WelcomeController extends Controller
{
public function getTable(Request $request)
{
if (!$request->ajax()) {
abort(404);
}
$count = $request->get('count');
$users = User::query()->limit($count)->get();
// 👇 table.blade.phpでhtmlを作って変数にセット
$table = view('table', [
'users' => $users
])->render();
return response()->json([
'table' => $table// 👈 レスポンスで作ったhtmlを返す
]);
}
}
📁 src/routes/web.php
Route::get('/table', [WelcomeController::class, 'getTable'])->name('table');
📁 src/resources/views/table.blade.php
<style>
table th, table td {
border: 1px solid black;
}
</style>
<table>
<thead>
<tr>
<th>
Id
</th>
<th>
Name
</th>
</tr>
</thead>
<tbody>
@foreach ($users as $user)
<tr>
<td>
{{$user->id}}
</td>
<td>
{{$user->name}}
</td>
</tr>
@endforeach
</tbody>
</table>
📁 src/resources/views/welcome.blade.php
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel</title>
<!-- 省略 -->
<div class="pt-3 sm:pt-5">
<h2 class="text-xl font-semibold text-black dark:text-white">Vibrant Ecosystem</h2>
<p class="mt-4 text-sm/relaxed">
Laravel's robust library of first-party tools and libraries, such as <a href="https://forge.laravel.com" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white dark:focus-visible:ring-[#FF2D20]">Forge</a>, <a href="https://vapor.laravel.com" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Vapor</a>, <a href="https://nova.laravel.com" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Nova</a>, <a href="https://envoyer.io" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Envoyer</a>, and <a href="https://herd.laravel.com" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Herd</a> help you take your projects to the next level. Pair them with powerful open source libraries like <a href="https://laravel.com/docs/billing" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Cashier</a>, <a href="https://laravel.com/docs/dusk" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Dusk</a>, <a href="https://laravel.com/docs/broadcasting" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Echo</a>, <a href="https://laravel.com/docs/horizon" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Horizon</a>, <a href="https://laravel.com/docs/sanctum" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Sanctum</a>, <a href="https://laravel.com/docs/telescope" class="rounded-sm underline hover:text-black focus:outline-none focus-visible:ring-1 focus-visible:ring-[#FF2D20] dark:hover:text-white">Telescope</a>, and more.
</p>
</div>
</div>
<!-- 👇 描画エリア -->
<div id="template_area">
</div>
<input type="number" name="count" value="0" style="border: 1px solid gray;">
<button type="button" id="get_template">render</button>
<button type="button" id="template_area_clear">clear</button>
<script src="https://code.jquery.com/jquery-3.7.1.js" integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4=" crossorigin="anonymous"></script>
<script>
$(function () {
$('#get_template').on('click', function (e) {
e.preventDefault();
$.ajax({
url: '/table',
type: 'GET',
dataType: 'json',
data: {
count: $('input[name="count"]').val()
},
success: function (data) {
$('#template_area').html(data.table);// 👈 描画処理
},
error: function (error) {
console.log(error);
}
});
});
$('#template_area_clear').on('click', function (e) {
e.preventDefault();
$('#template_area').html('');
});
});
</script>
</div>
</main>
<footer class="py-16 text-center text-sm text-black dark:text-white/70">
Laravel v{{ Illuminate\Foundation\Application::VERSION }} (PHP v{{ PHP_VERSION }})
</footer>
</div>
</div>
</div>
</body>
</html>