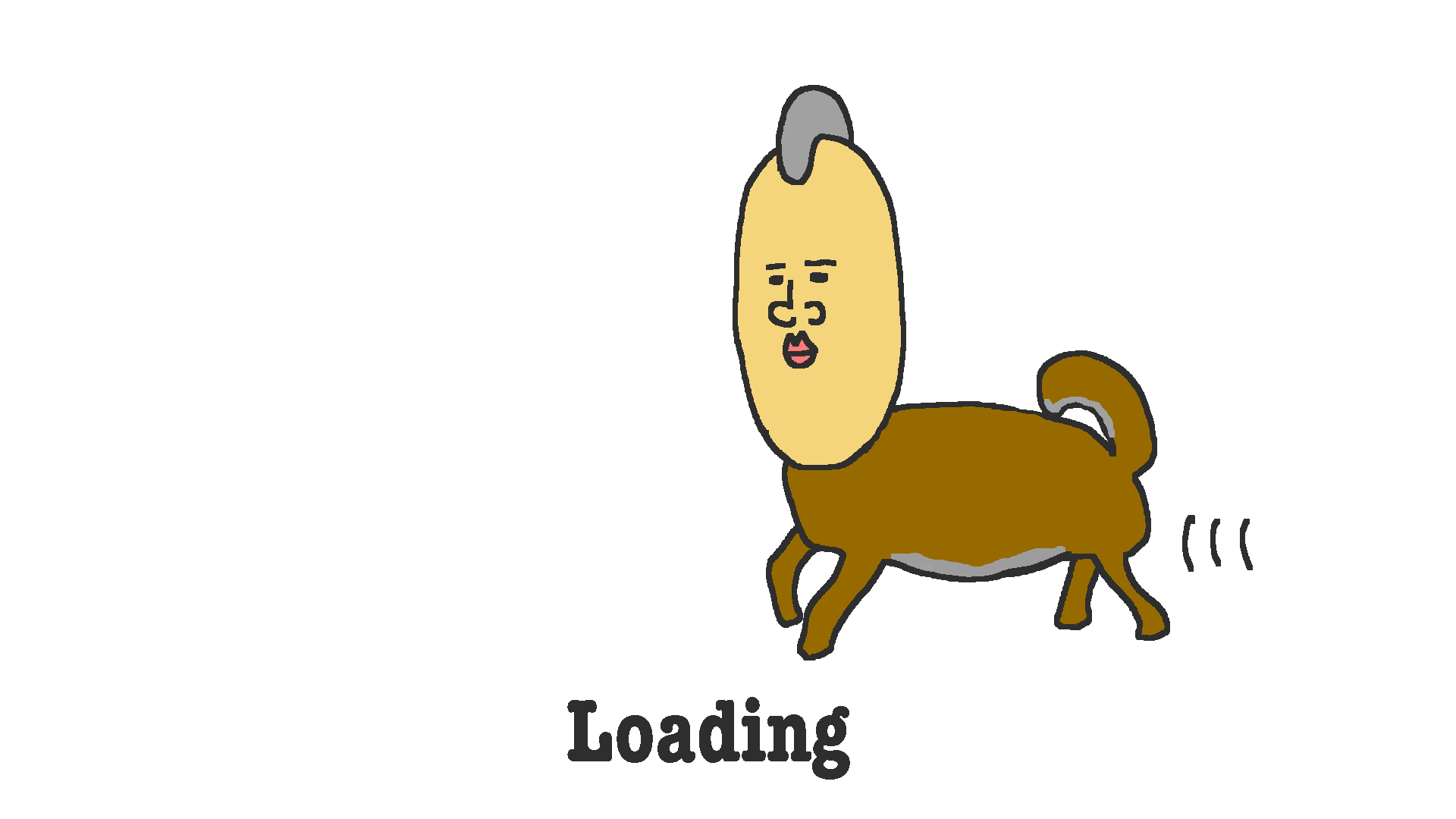
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
変更履歴
2021/09/12 validationのリダイレクト処理を編集しました。
php artisan make:notification PostArticleNotification
<?php namespace App\Notifications; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Notification; class PostArticleNotification extends Notification { use Queueable; /** * Create a new notification instance. * * @return void */ public function __construct() { // } /** * Get the notification's delivery channels. * * @param mixed $notifiable * @return array */ public function via($notifiable) { return ['mail']; } /** * Get the mail representation of the notification. * * @param mixed $notifiable * @return \Illuminate\Notifications\Messages\MailMessage */ public function toMail($notifiable) { return (new MailMessage) ->line('The introduction to the notification.') ->action('Notification Action', url('/')) ->line('Thank you for using our application!'); } /** * Get the array representation of the notification. * * @param mixed $notifiable * @return array */ public function toArray($notifiable) { return [ // ]; } }
//省略 use Illuminate\Support\Facades\Notification;//<-追加 use App\Notifications\PostArticleNotification;//<-追加 use App\User;//<-追加 //省略 public function create(Request $request) { $validator = Validator::make($request->all(), [ 'title' => [ 'required', 'string', 'max:25', ], 'content' => [ 'required', 'string', 'max:4000', ], 'tag' => [ 'nullable', 'string', ], ]); $validator->validate(); $id = $request->get('id'); $article = Article::find($id); if (isset($article) && $article->user_id !== Auth::user()->id) { abort(404); } $title = $request->get('title'); $content = $request->get('content'); if (isset($article)) { $article->title = $title; $article->content = $content; $article->save(); } else { $user_id = Auth::id(); $article = Article::create( [ 'title' => $title, 'content' => $content, 'user_id' => $user_id, ] ); } $input_tag = $request->get('tag'); if (isset($input_tag)) { $tag_ids = []; $tags = explode(',', $input_tag); foreach ($tags as $tag) { $tag = Tag::updateOrCreate( [ 'name' => $tag, ] ); $tag_ids[] = $tag->id; } $article->tags()->sync($tag_ids); } //ここから $users = User::where('id', '<>', $article->user->id)->get(); Notification::send($users, new PostArticleNotification()); //ここまで追加 return redirect()->route('article.show', ['id' => $article->id]); }
$users = User::where('id', '<>', $article->user->id)->get();
'<>'
'!='
Notification::send($users, new PostArticleNotification());
<?php namespace App; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; class User extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; }
//省略 use Illuminate\Notifications\Notifiable; //省略 use Notifiable; //省略
http://localhost:1080
$users = User::where('id', '<>', $article->user->id)->get(); Notification::send($users, new PostArticleNotification($article));//<-編集
<?php namespace App\Notifications; use App\Article; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Notifications\Messages\MailMessage; use Illuminate\Notifications\Notification; class PostArticleNotification extends Notification { use Queueable; private $article;//<-追加 /** * Create a new notification instance. * * @return void */ public function __construct( Article $article//<-追加 ) { $this->article = $article;//<-追加 } /** * Get the notification's delivery channels. * * @param mixed $notifiable * @return array */ public function via($notifiable) { return ['mail']; } /** * Get the mail representation of the notification. * * @param mixed $notifiable * @return \Illuminate\Notifications\Messages\MailMessage */ public function toMail($notifiable) { //↓諸々編集 return (new MailMessage) ->subject('ブログが新しく投稿されました!|' . config('app.name')) ->greeting($this->article->user->name . 'さんがブログを投稿しました!') ->line('title: ' . $this->article->title) ->action('ブログをみに行く', route('article.show', ['id' => $this->article->id])) ->line('アプリをご利用いただきありがとうございます。') ->salutation(config('app.name')); } /** * Get the array representation of the notification. * * @param mixed $notifiable * @return array */ public function toArray($notifiable) { return [ // ]; } }
private $article;
public function __construct( Article $article ) { $this->article = $article; }
__construct(){}
Article $article
$this->article = $article;
$this->プロパティ名
public function toMail($notifiable) { return (new MailMessage) ->subject('ブログが新しく投稿されました!|' . config('app.name')) ->greeting($this->article->user->name . 'さんがブログを投稿しました!') ->line('title: ' . $this->article->title) ->action('ブログをみに行く', route('article.show', ['id' => $this->article->id])) ->line('アプリをご利用いただきありがとうございます。') ->salutation(config('app.name')); }
config('app.name')
'name' => env('APP_NAME', 'Laravel'),
APP_NAME=Tutorial