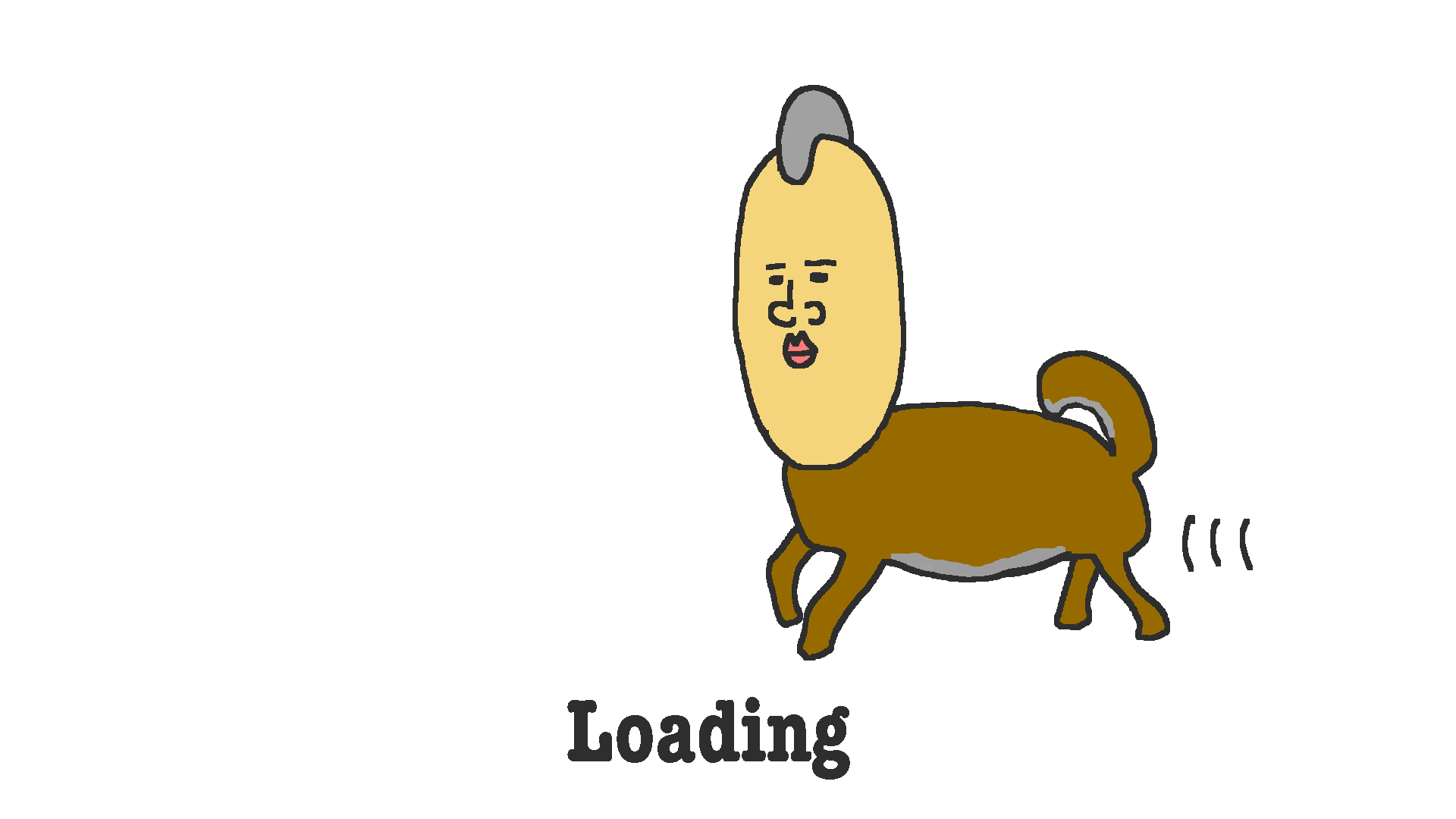
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
php artisan migrate
📁 vendor/laravel/framework/src/Illuminate/Database/Console/Migrations/MigrateCommand.php <?php namespace Illuminate\Database\Console\Migrations; use Illuminate\Console\ConfirmableTrait; use Illuminate\Database\Migrations\Migrator; class MigrateCommand extends BaseCommand { use ConfirmableTrait; /** * The name and signature of the console command. * * @var string */ protected $signature = 'migrate {--database= : The database connection to use} {--force : Force the operation to run when in production} {--path=* : The path(s) to the migrations files to be executed} {--realpath : Indicate any provided migration file paths are pre-resolved absolute paths} {--pretend : Dump the SQL queries that would be run} {--seed : Indicates if the seed task should be re-run} {--step : Force the migrations to be run so they can be rolled back individually}'; /** * The console command description. * * @var string */ protected $description = 'Run the database migrations'; /** * The migrator instance. * * @var \Illuminate\Database\Migrations\Migrator */ protected $migrator; /** * Create a new migration command instance. * * @param \Illuminate\Database\Migrations\Migrator $migrator * @return void */ public function __construct(Migrator $migrator) { parent::__construct(); $this->migrator = $migrator; } /** * Execute the console command. * * @return void */ public function handle() { if (! $this->confirmToProceed()) { return; } $this->prepareDatabase(); // Next, we will check to see if a path option has been defined. If it has // we will use the path relative to the root of this installation folder // so that migrations may be run for any path within the applications. $this->migrator->setOutput($this->output) ->run($this->getMigrationPaths(), [ 'pretend' => $this->option('pretend'), 'step' => $this->option('step'), ]); // Finally, if the "seed" option has been given, we will re-run the database // seed task to re-populate the database, which is convenient when adding // a migration and a seed at the same time, as it is only this command. if ($this->option('seed') && ! $this->option('pretend')) { $this->call('db:seed', ['--force' => true]); } } /** * Prepare the migration database for running. * * @return void */ protected function prepareDatabase() { $this->migrator->setConnection($this->option('database')); if (! $this->migrator->repositoryExists()) { $this->call('migrate:install', array_filter([ '--database' => $this->option('database'), ])); } } }
/** * The name and signature of the console command. * * @var string */ protected $signature = 'migrate ~ 省略 ~';
php artisan ~~~~ <-この部分
php artisan migrate
/** * Execute the console command. * * @return void */ public function handle() { if (! $this->confirmToProceed()) { return; } $this->prepareDatabase(); // Next, we will check to see if a path option has been defined. If it has // we will use the path relative to the root of this installation folder // so that migrations may be run for any path within the applications. $this->migrator->setOutput($this->output) ->run($this->getMigrationPaths(), [ 'pretend' => $this->option('pretend'), 'step' => $this->option('step'), ]); // Finally, if the "seed" option has been given, we will re-run the database // seed task to re-populate the database, which is convenient when adding // a migration and a seed at the same time, as it is only this command. if ($this->option('seed') && ! $this->option('pretend')) { $this->call('db:seed', ['--force' => true]); } }
$this->prepareDatabase();
/** * Prepare the migration database for running. * * @return void */ protected function prepareDatabase() { $this->migrator->setConnection($this->option('database')); if (! $this->migrator->repositoryExists()) { $this->call('migrate:install', array_filter([ '--database' => $this->option('database'), ])); } }
if (! $this->migrator->repositoryExists()) { $this->call('migrate:install', array_filter([ '--database' => $this->option('database'), ])); }
repositoryExists()
$this->call('migrate:install', ~~省略~~
repositoryExists()
📁 vendor/larave/framework/src/Illuminate/Database/Migrations/DatabaseMigrationRepository.php ~~省略~~ /** * Determine if the migration repository exists. * * @return bool */ public function repositoryExists() { $schema = $this->getConnection()->getSchemaBuilder(); return $schema->hasTable($this->table); } ~~省略~~
$schema = $this->getConnection()->getSchemaBuilder();
return $schema->hasTable($this->table);
hasTable($this->table);
php artisan migrate
📁 vendor/laravel/framework/src/Illuminate/Database/Console/Migrations/MigrateCommand.php ~~省略~~ if (! $this->migrator->repositoryExists()) { $this->call('migrate:install', array_filter([ '--database' => $this->option('database'), ])); } ~~省略~~
repositoryExists()
php artisan migrate
$this->call('migrate:install', array_filter([ '--database' => $this->option('database'), ]));
$this->call('~~~')
'migrate:install'
'migrate:install'
📁 vendor/laravel/framework/src/Illuminate/Database/Console/Migrations/InstallCommand.php <?php namespace Illuminate\Database\Console\Migrations; use Illuminate\Console\Command; use Illuminate\Database\Migrations\MigrationRepositoryInterface; use Symfony\Component\Console\Input\InputOption; class InstallCommand extends Command { /** * The console command name. * * @var string */ protected $name = 'migrate:install'; /** * The console command description. * * @var string */ protected $description = 'Create the migration repository'; /** * The repository instance. * * @var \Illuminate\Database\Migrations\MigrationRepositoryInterface */ protected $repository; /** * Create a new migration install command instance. * * @param \Illuminate\Database\Migrations\MigrationRepositoryInterface $repository * @return void */ public function __construct(MigrationRepositoryInterface $repository) { parent::__construct(); $this->repository = $repository; } /** * Execute the console command. * * @return void */ public function handle() { $this->repository->setSource($this->input->getOption('database')); $this->repository->createRepository(); $this->info('Migration table created successfully.'); } /** * Get the console command options. * * @return array */ protected function getOptions() { return [ ['database', null, InputOption::VALUE_OPTIONAL, 'The database connection to use'], ]; } }
/** * The console command name. * * @var string */ protected $name = 'migrate:install';
/** * Execute the console command. * * @return void */ public function handle() { $this->repository->setSource($this->input->getOption('database')); $this->repository->createRepository(); $this->info('Migration table created successfully.'); }
$this->repository->setSource($this->input->getOption('database'));
$this->repository->createRepository();
$this->info('Migration table created successfully.');
$this->repository->createRepository();
📁 vendor/laravel/framework/src/Illuminate/Database/Migrations/DatabaseMigrationRepository.php ~~省略~~ /** * Create the migration repository data store. * * @return void */ public function createRepository() { $schema = $this->getConnection()->getSchemaBuilder(); $schema->create($this->table, function ($table) { // The migrations table is responsible for keeping track of which of the // migrations have actually run for the application. We'll create the // table to hold the migration file's path as well as the batch ID. $table->increments('id'); $table->string('migration'); $table->integer('batch'); }); } ~~省略~~
$schema->create($this->table, function~~~
$this->table
'migrations'
$table->increments('id'); $table->string('migration'); $table->integer('batch');
📁 vendor/laravel/framework/src/Illuminate/Database/Console/Migrations/MigrateCommand.php ~~省略~~ /** * Execute the console command. * * @return void */ public function handle() { if (! $this->confirmToProceed()) { return; } $this->prepareDatabase(); // Next, we will check to see if a path option has been defined. If it has // we will use the path relative to the root of this installation folder // so that migrations may be run for any path within the applications. $this->migrator->setOutput($this->output) ->run($this->getMigrationPaths(), [ 'pretend' => $this->option('pretend'), 'step' => $this->option('step'), ]); // Finally, if the "seed" option has been given, we will re-run the database // seed task to re-populate the database, which is convenient when adding // a migration and a seed at the same time, as it is only this command. if ($this->option('seed') && ! $this->option('pretend')) { $this->call('db:seed', ['--force' => true]); } } ~~省略~~
// Next, we will check to see if a path option has been defined. If it has // we will use the path relative to the root of this installation folder // so that migrations may be run for any path within the applications. $this->migrator->setOutput($this->output) ->run($this->getMigrationPaths(), [ 'pretend' => $this->option('pretend'), 'step' => $this->option('step'), ]);
->run
repositoryExists()
php artisan migrate