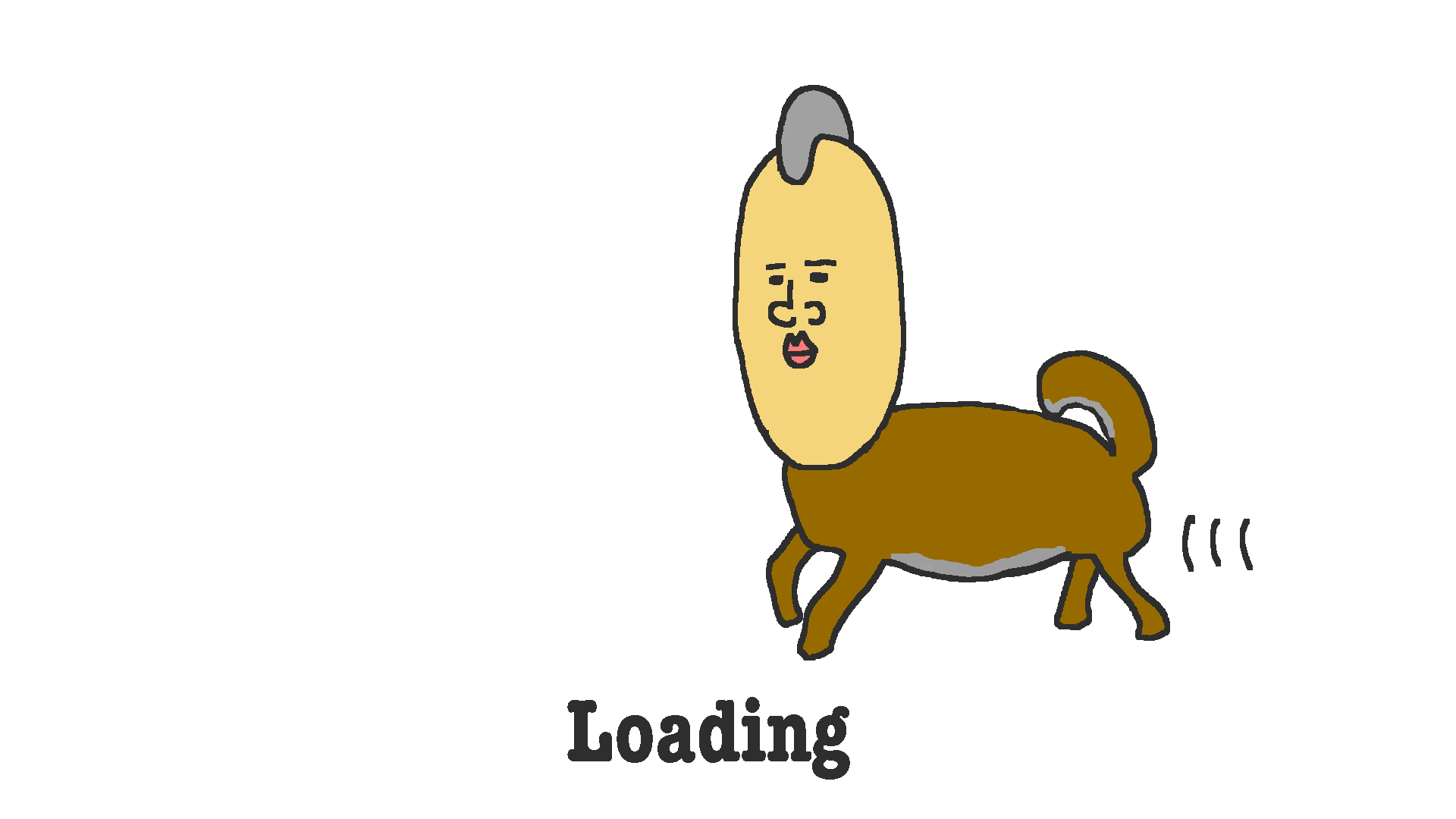
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
<?php namespace Customize\Controller\Mypage; use Eccube\Controller\AbstractController; use Eccube\Entity\Customer; use Symfony\Component\Security\Core\Encoder\EncoderFactoryInterface; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\Routing\Annotation\Route; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Template; use Eccube\Repository\CustomerRepository; class CheckCustomerPasswordController extends AbstractController { private $encoderFactory; private $customerRepository; public function __construct( EncoderFactoryInterface $encoderFactory, CustomerRepository $customerRepository ) { $this->encoderFactory = $encoderFactory; $this->customerRepository = $customerRepository; } /** * @param Request $request * @Route("/check/customer/password", name="check_customer_password", methods={"POST"}) * @Template("Hoge/huga.twig") */ public function checkCustomerPassword(Request $request) { $response = false; //フォームに入力されたemailとpasswordをrequestから取得 $email = $request->get('email'); $pass = $request->get('pass'); //requestのemailから登録済みのユーザーを検索 $customers = $this->customerRepository->createQueryBuilder('c') ->where('c.email = :email') ->setParameter('email', $email) ->getQuery() ->getResult(); //ユーザーがいたら if (!empty($customers)) { //CustomerEntityを取得 $customer = $customers[0]; //エンコーダーなるものを取得 $encoder = $this->encoderFactory->getEncoder($customer); //↓以下がパスワードがあっているか判定している if ($encoder->isPasswordValid($customer->getPassword(), $pass, $customer->getSalt())) { $response = true; } } return [ 'response' => $response, ]; } }
$customer = $this->customerRepository->findOneBy( [ 'email' => $email, ] ); if ($customer) { ...
20230406追記
ファイルのパスにAuthenticationの記述漏れがあったので追加しました
📁 /vender/symfony/security/Core/Authentication/Provider/DaoAuthenticationProvider.php <?php /* * This file is part of the Symfony package. * * (c) Fabien Potencier <fabien@symfony.com> * * For the full copyright and license information, please view the LICENSE * file that was distributed with this source code. */ namespace Symfony\Component\Security\Core\Authentication\Provider; use Symfony\Component\Security\Core\Authentication\Token\UsernamePasswordToken; use Symfony\Component\Security\Core\Encoder\EncoderFactoryInterface; use Symfony\Component\Security\Core\Exception\AuthenticationServiceException; use Symfony\Component\Security\Core\Exception\BadCredentialsException; use Symfony\Component\Security\Core\Exception\UsernameNotFoundException; use Symfony\Component\Security\Core\User\PasswordUpgraderInterface; use Symfony\Component\Security\Core\User\UserCheckerInterface; use Symfony\Component\Security\Core\User\UserInterface; use Symfony\Component\Security\Core\User\UserProviderInterface; /** * DaoAuthenticationProvider uses a UserProviderInterface to retrieve the user * for a UsernamePasswordToken. * * @author Fabien Potencier <fabien@symfony.com> */ class DaoAuthenticationProvider extends UserAuthenticationProvider { private $encoderFactory; private $userProvider; public function __construct(UserProviderInterface $userProvider, UserCheckerInterface $userChecker, string $providerKey, EncoderFactoryInterface $encoderFactory, bool $hideUserNotFoundExceptions = true) { parent::__construct($userChecker, $providerKey, $hideUserNotFoundExceptions); $this->encoderFactory = $encoderFactory; $this->userProvider = $userProvider; } /** * {@inheritdoc} */ protected function checkAuthentication(UserInterface $user, UsernamePasswordToken $token) { $currentUser = $token->getUser(); if ($currentUser instanceof UserInterface) { if ($currentUser->getPassword() !== $user->getPassword()) { throw new BadCredentialsException('The credentials were changed from another session.'); } } else { if ('' === ($presentedPassword = $token->getCredentials())) { throw new BadCredentialsException('The presented password cannot be empty.'); } if (null === $user->getPassword()) { throw new BadCredentialsException('The presented password is invalid.'); } $encoder = $this->encoderFactory->getEncoder($user); if (!$encoder->isPasswordValid($user->getPassword(), $presentedPassword, $user->getSalt())) { throw new BadCredentialsException('The presented password is invalid.'); } if ($this->userProvider instanceof PasswordUpgraderInterface && method_exists($encoder, 'needsRehash') && $encoder->needsRehash($user->getPassword())) { $this->userProvider->upgradePassword($user, $encoder->encodePassword($presentedPassword, $user->getSalt())); } } } /** * {@inheritdoc} */ protected function retrieveUser($username, UsernamePasswordToken $token) { $user = $token->getUser(); if ($user instanceof UserInterface) { return $user; } try { $user = $this->userProvider->loadUserByUsername($username); if (!$user instanceof UserInterface) { throw new AuthenticationServiceException('The user provider must return a UserInterface object.'); } return $user; } catch (UsernameNotFoundException $e) { $e->setUsername($username); throw $e; } catch (\Exception $e) { $e = new AuthenticationServiceException($e->getMessage(), 0, $e); $e->setToken($token); throw $e; } } }
/** * {@inheritdoc} */ protected function checkAuthentication(UserInterface $user, UsernamePasswordToken $token) { $currentUser = $token->getUser(); if ($currentUser instanceof UserInterface) { if ($currentUser->getPassword() !== $user->getPassword()) { throw new BadCredentialsException('The credentials were changed from another session.'); } } else { if ('' === ($presentedPassword = $token->getCredentials())) { throw new BadCredentialsException('The presented password cannot be empty.'); } if (null === $user->getPassword()) { throw new BadCredentialsException('The presented password is invalid.'); } $encoder = $this->encoderFactory->getEncoder($user); if (!$encoder->isPasswordValid($user->getPassword(), $presentedPassword, $user->getSalt())) { throw new BadCredentialsException('The presented password is invalid.'); } if ($this->userProvider instanceof PasswordUpgraderInterface && method_exists($encoder, 'needsRehash') && $encoder->needsRehash($user->getPassword())) { $this->userProvider->upgradePassword($user, $encoder->encodePassword($presentedPassword, $user->getSalt())); } } }
if (!$encoder->isPasswordValid($user->getPassword(), $presentedPassword, $user->getSalt())) { throw new BadCredentialsException('The presented password is invalid.'); }
getPassword() getSalt()
class Customer extends \Eccube\Entity\AbstractEntity implements UserInterface, \Serializable
isPasswordValid
$encoder = $this->encoderFactory->getEncoder($user);