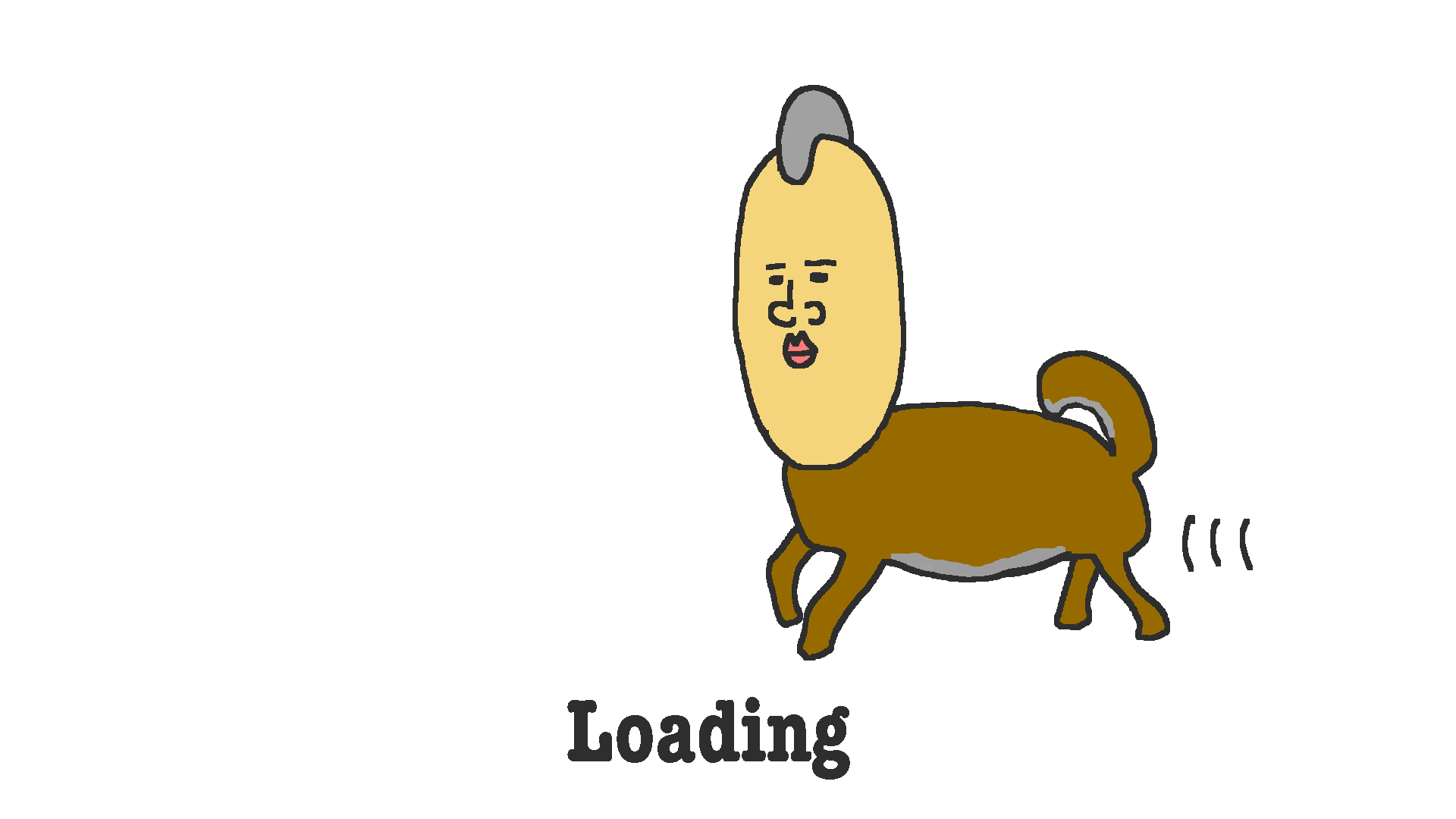
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
<?php namespace Eccube\Form\Type\Admin; // ..省略 use Eccube\Common\EccubeConfig; // ..省略 class OrderMailType extends AbstractType { /** * @var EccubeConfig */ protected $eccubeConfig; /** * MailType constructor. * * @param EccubeConfig $eccubeConfig */ public function __construct( EccubeConfig $eccubeConfig ) { $this->eccubeConfig = $eccubeConfig; } /** * {@inheritdoc} */ public function buildForm(FormBuilderInterface $builder, array $options) { $builder ->add('template', MailTemplateType::class, [ 'required' => false, 'mapped' => false, 'query_builder' => function (EntityRepository $er) { return $er->createQueryBuilder('mt') ->andWhere('mt.id = :id') // 👇こいつ ->setParameter('id', $this->eccubeConfig['eccube_order_mail_template_id']) ->orderBy('mt.id', 'ASC'); }, ]) // ..省略
->setParameter('id', $this->eccubeConfig['eccube_order_mail_template_id'])
<?php /* * This file is part of EC-CUBE * * Copyright(c) EC-CUBE CO.,LTD. All Rights Reserved. * * http://www.ec-cube.co.jp/ * * For the full copyright and license information, please view the LICENSE * file that was distributed with this source code. */ namespace Eccube\Common; use Symfony\Component\DependencyInjection\ContainerInterface; // 👇これ class EccubeConfig implements \ArrayAccess { /** * @var ContainerInterface */ protected $container; public function __construct(ContainerInterface $container) { $this->container = $container; } /** * @param $key * * @return mixed */ public function get($key) { return $this->container->getParameter($key); } /** * @param $key * * @return bool */ public function has($key) { return $this->container->hasParameter($key); } /** * @param $key * @param $value */ public function set($key, $value) { $this->container->setParameter($key, $value); } /** * @param mixed $offset * * @return bool */ #[\ReturnTypeWillChange] public function offsetExists($offset) { return $this->has($offset); } /** * @param mixed $offset * * @return mixed */ #[\ReturnTypeWillChange] public function offsetGet($offset) { return $this->get($offset); } /** * @param mixed $offset * @param mixed $value */ #[\ReturnTypeWillChange] public function offsetSet($offset, $value) { $this->set($offset, $value); } /** * @param mixed $offset * * @throws \Exception */ #[\ReturnTypeWillChange] public function offsetUnset($offset) { throw new \Exception(); } }
📁 Obj.php <?php class Obj implements ArrayAccess { private $container = array(); public function __construct( array $array ) { $this->container = $array; } public function offsetSet($offset, $value) { if (is_null($offset)) { $this->container[] = $value; } else { $this->container[$offset] = $value; } } public function offsetExists($offset) { return isset($this->container[$offset]); } public function offsetUnset($offset) { unset($this->container[$offset]); } public function offsetGet($offset) { return isset($this->container[$offset]) ? $this->container[$offset] : null; } }
📁 test.php <?php require_once ('./Obj.php'); $array = [ "one" => 1, "two" => 2, "three" => 3, ]; $obj = new Obj($array); var_dump($obj['test']); $obj['test'] = 'osu'; var_dump($obj['test']); var_dump($obj['one']);
NULL string(3) "osu" int(1)