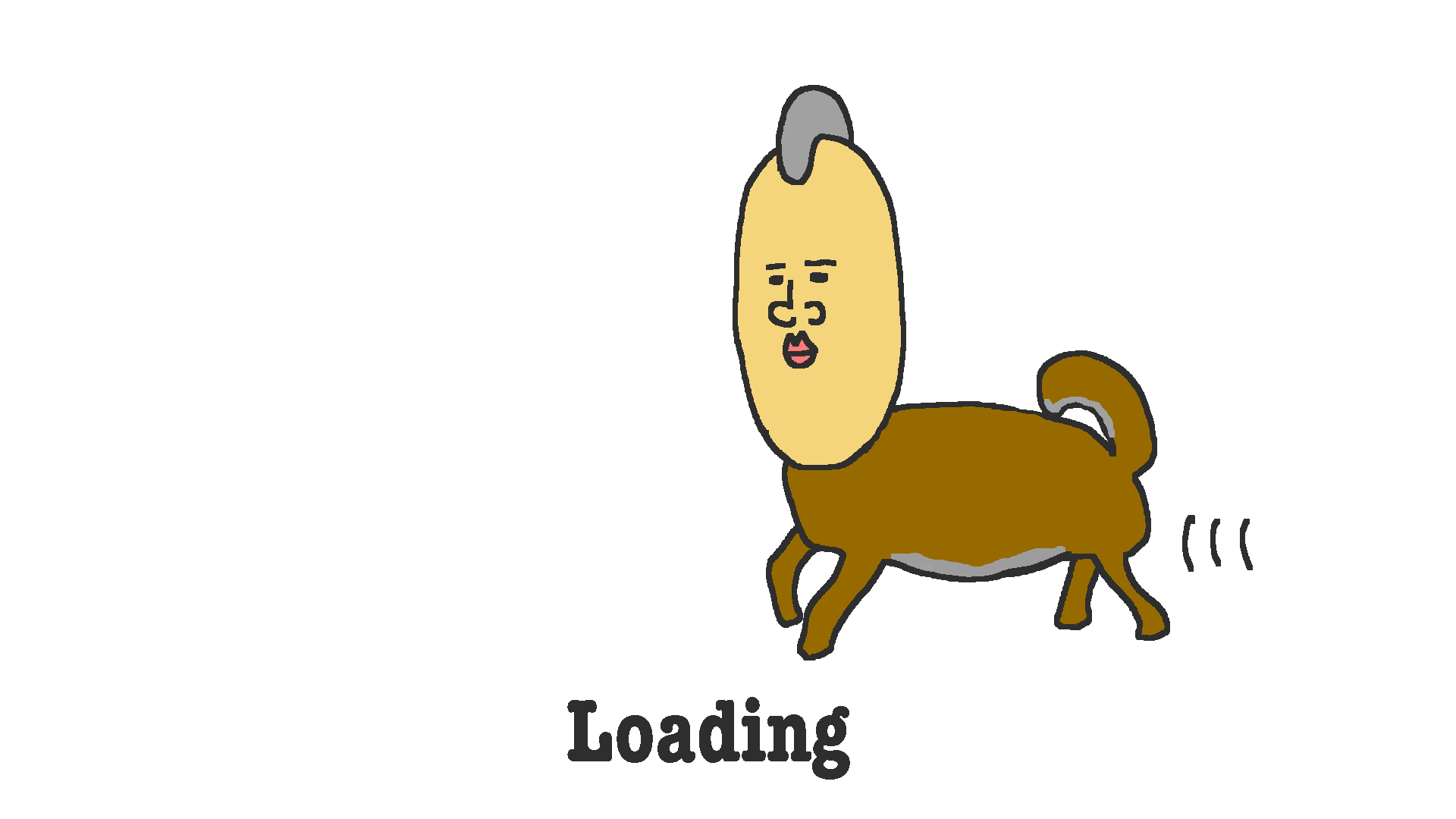
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
📁 root/app/User.php <?php namespace App; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use App\Article; class User extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; // 👇 リレーション定義 public function articles() { return $this->hasMany(Article::class); } }
📁 root/app/Http/Controllers/UserController.php <?php namespace App\Http\Controllers; use App\Http\Controllers\Controller; use App\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class UserController extends Controller { public function index(Request $request) { $user_id = Auth::id(); $user = User::query() ->where('id', '=', $user_id) ->with(['articles'])// 👈 これ ->first(); return view( 'user.index', [ 'user' => $user, ] ); } }
📁 root/routes/web.php // ~~ 省略 Route::get('/user/index', 'UserController@index')->name('user.index');
📁 root/resources/views/user/index.blade.php @extends('layouts.app') @section('content') @foreach ($user->articles as $article) <div> user_name: {{$user->name}} </div> <div style="border-bottom: solid 1px gray;"> <div> title: <a href="{{route('article.show', ['id' => $article->id])}}">{{$article->title}}</a> <small>{{$article->created_at}}</small> </div> </div> @endforeach @endsection
📁 root/app/Http/Controllers/UserController.php <?php namespace App\Http\Controllers; use App\Http\Controllers\Controller; use App\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class UserController extends Controller { public function index(Request $request) { $user_id = Auth::id(); $user = User::query() ->where('id', '=', $user_id) // 👇 修正 ->with(['articles' => function ($query) { $query->orderByDesc('id'); }]) ->first(); return view( 'user.index', [ 'user' => $user, ] ); } }
->with(['articles' => function ($query) {
$query->get();
$query->orderByDesc('id');
📁 root/app/User.php // ~~省略 public function articles() { return $this->hasMany(Article::class) ->orderBy('id');// 👈 これ }