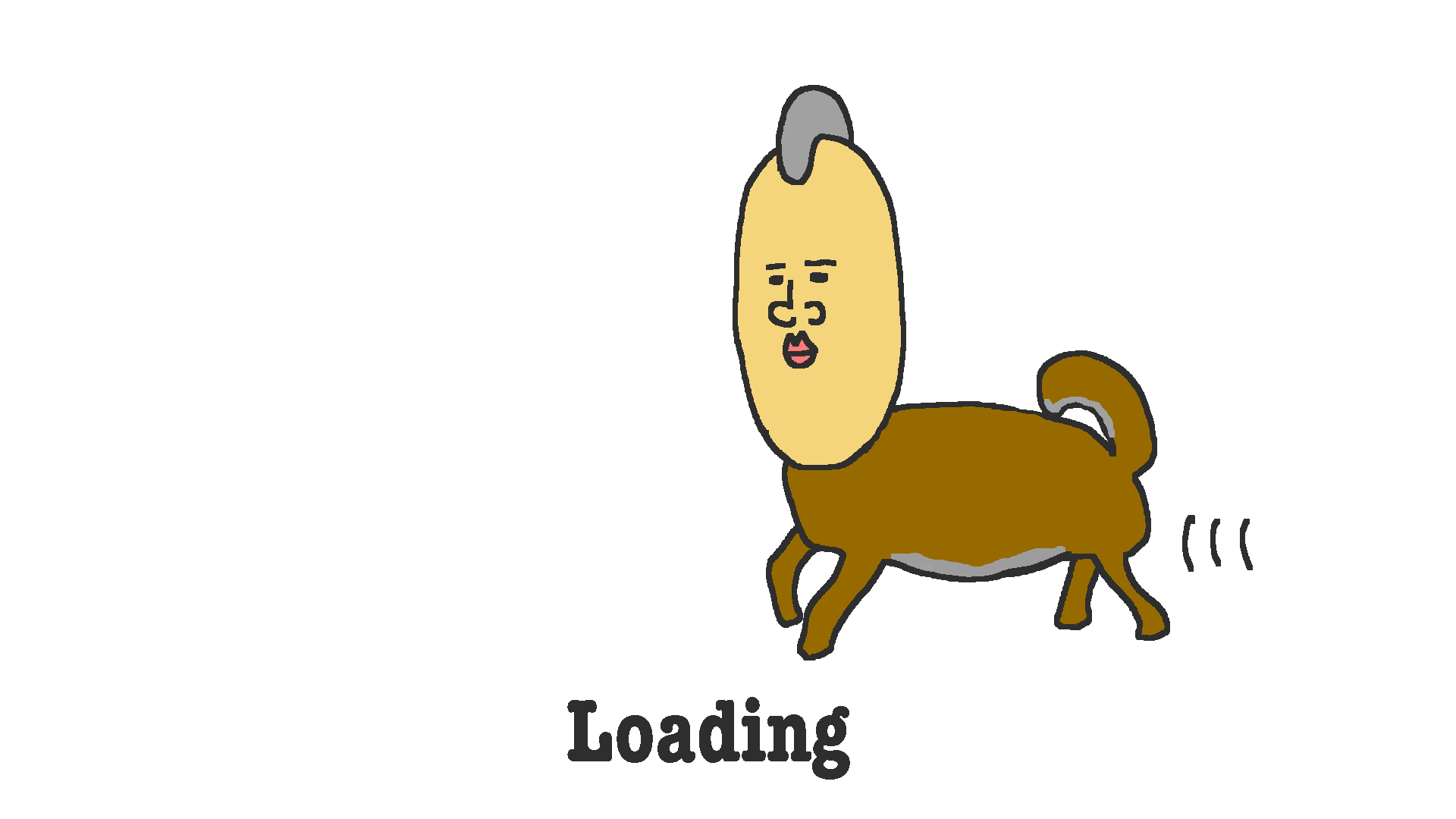
読み込みが終了しない場合は、しばらく待つか、リロードを行なってください。
If loading does not finish, wait for a while or reload.
エンジニア向けの情報を発信するブログです。
どなたでも発信できます。
お好きに利用していただれば幸いです。
📁 app/Customize/Repository/Extension/ProductRepositoryExtension.php <?php namespace Customize\Repository\Extension; use Eccube\Repository\ProductRepository; use Eccube\Repository\QueryKey; use Eccube\Util\StringUtil; class ProductRepositoryExtension extends ProductRepository { /** * get query builder. * * @param array $searchData * * @return \Doctrine\ORM\QueryBuilder */ public function getQueryBuilderBySearchData($searchData) { $qb = $this->createQueryBuilder('p') ->andWhere('p.Status = 1'); // category $categoryJoin = false; if (!empty($searchData['category_id']) && $searchData['category_id']) { $Categories = $searchData['category_id']->getSelfAndDescendants(); if ($Categories) { $qb ->innerJoin('p.ProductCategories', 'pct') ->innerJoin('pct.Category', 'c') ->andWhere($qb->expr()->in('pct.Category', ':Categories')) ->setParameter('Categories', $Categories); $categoryJoin = true; } } // name if (isset($searchData['name']) && StringUtil::isNotBlank($searchData['name'])) { $keywords = preg_split('/[\s ]+/u', str_replace(['%', '_'], ['\\%', '\\_'], $searchData['name']), -1, PREG_SPLIT_NO_EMPTY); foreach ($keywords as $index => $keyword) { $key = sprintf('keyword%s', $index); $qb ->andWhere(sprintf('NORMALIZE(p.name) LIKE NORMALIZE(:%s) OR NORMALIZE(p.search_word) LIKE NORMALIZE(:%s) OR EXISTS (SELECT wpc%d FROM \Eccube\Entity\ProductClass wpc%d WHERE p = wpc%d.Product AND NORMALIZE(wpc%d.code) LIKE NORMALIZE(:%s))', $key, $key, $index, $index, $index, $index, $key)) ->setParameter($key, '%'.$keyword.'%'); } } // Order By // 価格低い順 $config = $this->eccubeConfig; if (!empty($searchData['orderby']) && $searchData['orderby']->getId() == $config['eccube_product_order_price_lower']) { //@see http://doctrine-orm.readthedocs.org/en/latest/reference/dql-doctrine-query-language.html // 👇書き換え $qb->addSelect('(CASE WHEN p.id = :one THEN 1 WHEN p.id = :three THEN 2 WHEN p.id = :two THEN 3 ELSE 9999 END) AS HIDDEN CUSTOME_ORDER') ->setParameter('one', 1) ->setParameter('three', 3) ->setParameter('two', 2); $qb->innerJoin('p.ProductClasses', 'pc'); $qb->andWhere('pc.visible = true'); $qb->groupBy('p.id'); // 👇書き換え $qb->orderBy('CUSTOME_ORDER', 'ASC'); $qb->addOrderBy('p.id', 'DESC'); // 価格高い順 } elseif (!empty($searchData['orderby']) && $searchData['orderby']->getId() == $config['eccube_product_order_price_higher']) { // 👇書き換え $qb->addSelect('(CASE WHEN p.id = :three THEN 1 WHEN p.id = :one THEN 2 WHEN p.id = :two THEN 3 ELSE 9999 END) AS HIDDEN CUSTOME_ORDER') ->setParameter('one', 1) ->setParameter('three', 3) ->setParameter('two', 2); $qb->innerJoin('p.ProductClasses', 'pc'); $qb->andWhere('pc.visible = true'); $qb->groupBy('p.id'); // 👇書き換え $qb->orderBy('CUSTOME_ORDER, p.id'); $qb->addOrderBy('p.id', 'DESC'); // 新着順 } elseif (!empty($searchData['orderby']) && $searchData['orderby']->getId() == $config['eccube_product_order_newer']) { // 在庫切れ商品非表示の設定が有効時対応 // @see https://github.com/EC-CUBE/ec-cube/issues/1998 if ($this->getEntityManager()->getFilters()->isEnabled('option_nostock_hidden') == true) { $qb->innerJoin('p.ProductClasses', 'pc'); $qb->andWhere('pc.visible = true'); } $qb->orderBy('p.create_date', 'DESC'); $qb->addOrderBy('p.id', 'DESC'); } else { if ($categoryJoin === false) { $qb ->leftJoin('p.ProductCategories', 'pct') ->leftJoin('pct.Category', 'c'); } $qb ->addOrderBy('p.id', 'DESC'); } return $this->queries->customize(QueryKey::PRODUCT_SEARCH, $qb, $searchData); } }
// ~~省略 $qb->addSelect('(CASE WHEN p.id = :three THEN 1 WHEN p.id = :one THEN 2 WHEN p.id = :two THEN 3 ELSE 9999 END) AS HIDDEN CUSTOME_ORDER') ->setParameter('one', 1) ->setParameter('three', 3) ->setParameter('two', 2); // ~~ 省略 $qb->orderBy('CUSTOME_ORDER', 'ASC');
$qb->orderBy('CUSTOME_ORDER, p.id');